Differences Between Byte Code, Assembly Code, and Machine Code
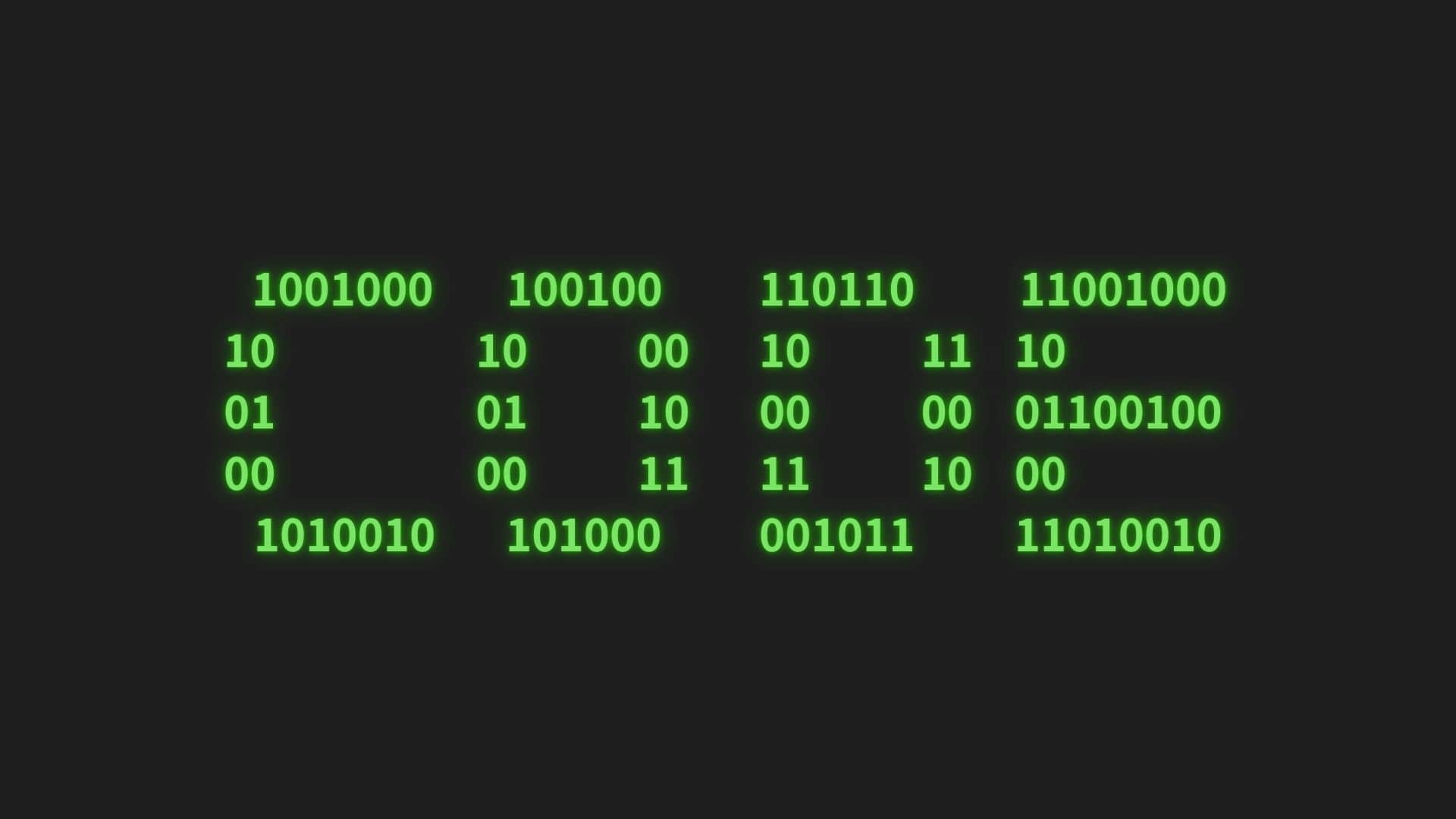
Differences Between Byte Code, Assembly Code, and Machine Code
CX330Disclaimer ⚠️
These contents are generated by ChatGPT and are intended for my personal review only. The accuracy of the contents is not guaranteed.
Byte Code
- Definition: Byte code is an intermediate code form that is closer to machine language than source code but still independent of the specific hardware platform. It is typically generated by compilers of high-level languages and executed by virtual machines.
- Characteristics:
- Platform Independence: Byte code can run on different hardware platforms as long as there is a corresponding virtual machine (e.g., JVM or Python Virtual Machine).
- Higher Abstraction Level: Closer to the structure of high-level languages compared to machine code.
- Requires Virtual Machine: Byte code is not executed directly by hardware but is interpreted or further compiled by virtual machines (e.g., Java Virtual Machine or Python Interpreter).
- Examples: Compiled Java
.class
files or compiled Python.pyc
files.
Assembly Code
Definition: Assembly code is a low-level programming language that uses mnemonics to represent machine instructions, typically associated with a specific computer architecture.
Characteristics:
- Human-readable: Easier to read and understand than machine code, but still relatively difficult.
- Direct Correspondence to Machine Instructions: Each assembly instruction usually corresponds directly to a machine instruction.
- Hardware Dependent: Assembly code is closely related to specific processor architectures (e.g., x86, ARM) and requires an assembler to convert it into machine code.
Examples: A simple x86 assembly code example for adding two numbers and returning the result:
section .data num1 db 10 num2 db 20 section .text global _start _start: mov al, [num1] add al, [num2] mov [result], al
Machine Code
- Definition: Machine code is the binary code that a computer’s CPU can directly understand and execute, consisting of binary digits (0 and 1).
- Characteristics:
- Lowest-level Code: Made up of 0s and 1s, it is the most fundamental level of code that hardware can directly execute.
- Hardware Dependent: Each processor architecture has its own set of machine instructions, making machine code completely hardware-specific.
- Most Difficult to Read: Machine code is the hardest form of code for humans to understand, but it is the fastest to execute because it is directly executed by the hardware.
- Examples: Machine code looks like a sequence of binary data:
11001000 10110001 00000001 11000011
Summary
Byte Code:
- Usage: Enables the same program to run on different platforms through virtual machines.
- Execution: Interpreted or JIT-compiled by the virtual machine.
- Languages: Java, Python, etc.
Assembly Code:
- Usage: Provides precise control over hardware, used for writing high-performance or system-level code.
- Execution: Needs to be assembled into machine code by an assembler, then executed by the CPU.
- Languages: Used in system programming and other low-level applications.
Machine Code:
- Usage: Direct instructions executed by computer hardware.
- Execution: Directly executed by the CPU.
- Languages: The final output of compilers and assemblers for languages like C, Rust, etc.
Comment
Privacy policy
✅ No need to delete blank lines, comment directly for the best display